Unraveling Cryptography: 7 Essential Crypto Concepts Every Developer Must Know
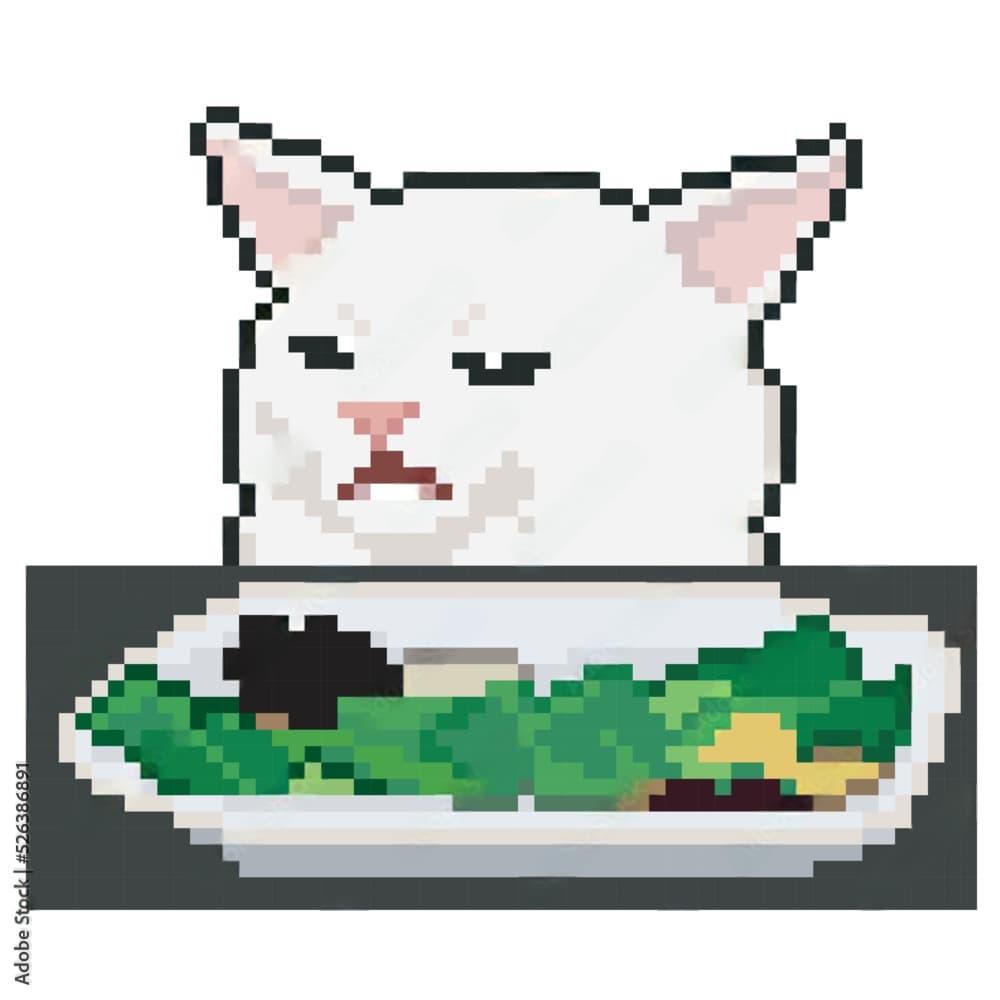
Written by Massa Medi
Cryptography is terrifying. Let’s be honest—most of us are a little intimidated by it. It’s rooted in arcane mathematics that make even the bravest developer break into a cold sweat. Yet, cryptography isn’t just for digital wizards—it’s the silent defender that keeps our online world secure. Without the mysterious art of cryptography, the Internet would be a hacker’s playground: your emails, bank records, and even your innocent browser history would be ripe for the picking.
See, computers do exactly what we tell them. But sometimes what we don’t want them to do is expose our private data. Picture the classic sci-fi line: “I’m sorry, Dave, I’m afraid I can’t do that.”—except, in this universe, it’s cryptography keeping your secrets safe, not HAL 9000.
At its core, cryptography scrambles data using algorithms, making it nearly impossible to make sense of anything without the proper credentials. Today, we’re diving into seven powerful concepts every developer should understand—complete with hands-on Node.js code so you can follow along. And, as a little incentive: stay until the end for a classic developer challenge (with a free Lifetime Pro membership and T-shirt up for grabs for the first solver).
The Ancient Roots—and Rapid Evolution—of Cryptography
Cryptography predates computers by millennia. Ancient humans had creative—if somewhat unfortunate—methods for safeguarding secrets. For example, they’d tattoo confidential messages on a slave’s shaved scalp, then let the hair regrow to hide the message. The glaring flaw? All an attacker had to do was shave the head again. Not exactly foolproof.
By the time of Julius Caesar, things got at least a little bit smarter. The Caesar cipher replaced each letter with another, shifted by a fixed amount in the alphabet—a huge leap in secret communication at the time.
Fast-forward to the near future—say, 2025—and imagine quantum computers reducing today’s cutting-edge crypto algorithms to ancient relics. The point? Cryptography never stands still. What’s secure now could be tomorrow’s punchline.
But don’t panic. For most developers, it’s not essential to master all the math underpinning cryptography. What is critical: understanding the core concepts—like hashing, encryption, and digital signatures—that underpin modern digital security.
1. Hashing: Chopping, Mixing, Obscuring
The word “hash” can be intimidating—but if you’re hungry, think of “hash” as a culinary technique: chop and mix. That’s pretty much what a hash function does to your data! You start with an input of any length (could be a password, a file, or even a novel). Pass it to a hashing function, and you’ll always get back a fixed-length, meaningless-looking string (the hash).
- Deterministic: The same input always yields the same output.
- Fast to compute: Efficiency matters for real applications.
- Unique: It’s extremely hard (in theory) for two different inputs to produce the same hash.
- One-way: Going from hash back to input is (almost) impossible.
Why is this useful? For starters, you’ll use hashes for things like passwords. You don't want to store raw passwords in your database! If a hacker steals the database, all bets are off. Hash values, on the other hand, offer an extra layer of protection. But even that’s not foolproof, as we’ll see.
Implementing Hashing in Node.js
To try this yourself, all you need is Node.js. Here’s a step-by-step walkthrough:
- Import the
createHash
function from Node’scrypto
module. - Define your hashing function.
- Choose your algorithm. This example uses SHA256 (“Secure Hash Algorithm 256-bit”). For curious minds: SHA256 produces hashes 256 bits in length.
- Hash your input: Feed in your string, and output a hexadecimal result (that’s a classic format, though
base64
works too).
Pro Tip: Avoid MD5! It’s obsolete—check out SHA256 or even Argon2 (though the latter isn’t included out-of-the-box in Node’s crypto module).
What does the output look like? Imagine passing "password" to your function—what you get is a unique, bewildering string that obscures the original input. When you hash the same password again, you’ll get the same hash—perfect for later comparison.
But: Hashing alone isn’t enough for password security. That brings us to "salting."
2. Salt: Spicing Up Your Security
A hashing function always returning the same value is problematic—especially when humans pick weak passwords like “password123.” (Or, borrowing from popular culture: "The combination is 1, 2, 3, 4, 5? That’s the stupidest combination I ever heard in my life!").
If a hacker gets their hands on your database, and you haven’t salted your hashes, they’ll simply use precomputed “rainbow tables” to look up the original passwords. Salting to the rescue!
What’s a salt? It’s a random value added to your password before hashing, making each hash unique even if two users have the same password.
Salting & Hashing in Node.js
For next-level protection, you’ll want to use Node’s scrypt
function (also from the crypto
module) plus randomBytes
to generate your salts. Here’s the general flow:
- On user sign-up, generate a random salt.
- Hash the password + salt using
scrypt
, specifying a sufficiently large key length (64 bytes is recommended). - Store both the hash and the salt in your database (e.g., concatenate with a separator).
- On login, retrieve the salt, re-hash the entered password using that salt, and compare.
Scrypt isn’t just hard to brute force—it’s also used in some cryptocurrency mining algorithms!
Security tip: Use Node’s timingSafeEqual
function to compare hashes. Traditional string comparison leaks timing info that sophisticated attackers could exploit.
3. HMAC: Hashes With a Secret Ingredient
Next up: HMAC, or Hash-based Message Authentication Code. An HMAC is a hash that also requires a secret password (or key). The result? Only someone who knows the secret can produce or verify the HMAC.
Real-world example: JSON Web Tokens (JWT) for web authentication. The server creates a token signed with a secret; if you try to forge it without that secret, no dice.
HMAC in Node.js
Use the createHmac
function, supply your secret, and create a hash of your message. The key difference from regular hash? The same password (secret) is needed to produce and verify the hash signature.
- Matching secrets: Same input and same key = identical HMAC.
- Different secrets: Even with the same message, a different key = entirely different HMAC.
4. Encryption: Scrambling Secrets (and Unscrambling Them)
What’s encryption? You take a message, scramble it so it’s unreadable (ciphertext), but—unlike hashing—you (or someone trusted) can later unscramble it if you have the right key.
A key point: Encryption is usually randomized, so encrypting the same message twice yields completely different ciphertexts. That makes it way harder for hackers to analyze!
Symmetric Encryption in Node.js
Symmetric encryption means the same password (key) is used for scrambling and unscrambling. Here’s how you do it in Node.js:
- Import
createCipheriv
andcreateDecipheriv
, plusrandomBytes
. - Generate a key (e.g., 32 random bytes for AES-256) and an initialization vector (IV, 16 random bytes). The IV randomizes the output, so repeating the same message doesn’t create the same ciphertext.
- Encrypt: Use the cipher’s
update
andfinal
methods to process your data into ciphertext. - Decrypt: Create a decipher object (with the same key and IV), and reverse the process.
Analogy: Think of data as a secret message in a locked box. Both the sender and receiver need a copy of the same key to unlock the box. Great for small groups, but what if you need to send a secret to someone you’ve never met before?
5. Public-Key Cryptography (Asymmetric Encryption): Mailboxes for the Digital Age
Symmetric encryption’s weakness? Both parties need to agree on a shared secret. That’s not practical for strangers!
Here’s where public-key cryptography shines. Every user has two keys:
- Public key: Freely shareable. Like your mailbox’s slot—anyone can slip mail in.
- Private key: Keep this secret! It opens the mailbox to retrieve messages.
In Node.js, generate these with generateKeyPair
. The most common system is RSA, and options like key length and encoding add flexibility and security.
Tip: For extra security, you can add a passphrase to your private key.
How does this protect you online? Any time you visit a website over HTTPS, your browser fetches that site’s public key from its SSL certificate. Anything you type (your secret) is encrypted with that public key—only the server (with its private key) can decrypt it.
Asymmetric Encryption in Node.js
Grab publicEncrypt
and privateDecrypt
from Node’s crypto module. Combine the public key with your message (as a buffer) and voilà: an encrypted message the recipient (and only the recipient) can unlock.
6. Digital Signatures: Proving It’s Really You
Sometimes, encryption isn’t about hiding information but proving authenticity. Enter the digital signature.
Imagine sending a physical letter stuffed with sensitive info. How do you guarantee it came from the right sender—and hasn’t been tampered with in transit? Traditionally, you might use a wax seal, or even a signature in blood if you’re feeling dramatic. In the digital world, we do the same, minus the biohazard.
How do digital signatures work?
- The sender hashes the original message.
- They use their private key to sign that hash, creating a unique signature.
- The recipient can use the sender’s public key to verify that the signature matches and hasn’t been forged or altered.
Signing in Node.js
Use Node’s createSign
function with an algorithm like RSASSA-PKCS1-v1_5 with SHA256 for hashing. After signing, attach the signature to your message. The recipient, using createVerify
and the sender’s public key, can confidently confirm authenticity and integrity.
If the signature fails verification, your message has been forged or tampered with—just like a broken wax seal!
7. The Ultimate Developer Challenge: Crack the Hash
That’s seven cryptographic superpowers every developer should have in their toolkit. But before you close this tab, here’s your challenge:
In the GitHub repo, there’s a file called hack.js
. Inside it: a hash. Use your skills to crack it and submit your answer as a pull request in the repo. First solver scores a free Lifetime Pro membership and T-shirt.
So! If this hands-on guide helped demystify cryptography (or even inspired you to finally use a unique password), hit that like button, subscribe, and—most importantly—remember: in a world run by little ones and zeros, knowledge is the best defense.
Thanks for reading, and see you for the next deep dive!